The purpose of this post is to create the starting project for the web service backend.
Later, we will create the augmented reality Windows 8 client. 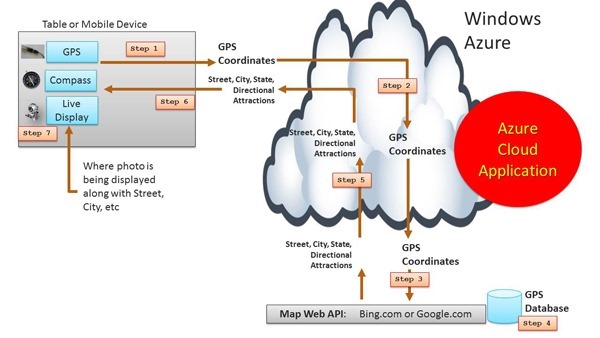
- Let's start with the cloud back-end.
- The mobile application will send the Azure Cloud Application GPS coordinates.
- The Cloud Application will use the coordinates to do a lookup at Google.com or Bing.com to find more information about those coordinates.
- For example, it can lookup the city and neighborhood.
- The Cloud application will return this data back to the client for display on the screen, overlaying the photo.
Notice that we are selecting an MVC Web API application.

- It is important to understand WHY I am choosing an MVC Web API project type.
- There are basically two options to build the web services project:
- Use Windows Communication Foundation - WCF -, or
- Use ASP.NET Web API, which is included with MVC version 4.
- Exposing services via WCF is also easy to do.
- But for this specific scenario we will use the newer, more modern approach that ASP.NET Web API brings to the table, truly embracing HTTP concepts (URIs and verbs).
- The MVC Framework allows us to create services that use more easily use some HTTP features, such as request/response headers, along with hypermedia constructs.
- Both projects can be tested on a single machine during development.
- Watch the following 1.5 minute video to see how to create a cloud-based MVC4 application.
Understanding Role Types for a Windows Azure Project - What the options mean
- ASP.NET Web Role
- This is old school web forms.
- This is a very well-established pattern for creating modern web applications.
- There is a well established eco-system of tools, documentation and resources.
- But it doesn't lend itself to unit testing.
- It doesn't have a clear separation of code-behind and markup.
- Many developers have moved on to ASP.NET MVC, described next.
- ASP.NET MVC3/4 Web Role (what we choose in these posts)
- This is the popular and powerful framework from Microsoft.
- It is a web application framework that implements the model-view-controller (MVC) pattern.
- It also provides the ability throu the Web API to create REST-based web services. -We will choose this option.
- Can do unit testing and has clear separation of concerns.
- WCF Service Web Role
- The Windows Communication Foundation (or WCF) is a runtime and a set of APIs in the .NET Framework for building connected, service-oriented applications.
- It has been used heavily for SOA-type applications, but has given ground to the Web API as REST-based architectures grew in popularity.
- This is a perfectly acceptable solution, and the only solution, if you need to support many advanced Web services (WS) standards such as WS-Addressing, WS-ReliableMessaging and WS-Security.
- With subsequent versions of the NET Framework 4.0, WCF also provides RSS Syndication Services, WS-Discovery, routing and better support for REST services.
- Worker Role
- So far we have been taking about web roles. Web roles, by default, include Internet Information Server (IIS) inside of a Windows Server OS inside a VM, running on one physical core.
- Worker roles are the same thing as web roles, except there is no IIS.
- This allows cloud-based applications to run background processes, typically reading messages placed in queues by web roles.
- Worker roles can leverage Windows Azure Storage, just like web roles.
- Cache Worker Role
- Windows Azure Caching supports the ability to host Caching services on Windows Azure roles.
- In this model, the cache can join memory resources to form a cache cluster.
- This private cache cluster is available only to the roles within the same deployment.
- Your application is the only consumer of the cache.
- There are no predefined quotas or throttling.
- Physical capacity (memory and other physical resources) is the only limiting factor.
- Other features include named caches, regions, tagging, high availability, local cache with notifications, and greater API symmetry with Microsoft AppFabric 1.1 for Windows Server.
Understanding VisualController is a key starting point
- VisualController.cs is a file we need to modify.
- It contains the code that will execute when the Windows 8 client submits an HTTP request against the web service.
- This HTTP request will include GPS data.
- This is where we will add some of our code to return the JSON data required by the Windows 8 application.
- The ValuesController class is generated by Visual Studio, and it inherits from ApiController, which returns data that is serialized and sent to the client, automatically in JSON format.
- We will test this file before modifying it. We need to learn how to call methods inside of VisualController.cs
|
123456789101112131415161718192021222324252627282930 |
public class ValuesController : ApiController{ // GET api/values public IEnumerable<string> Get() { return new string[] { "value1", "value2" }; } // GET api/values/5 public string Get(int id) { return "value"; } // POST api/values public void Post(string value) { } // PUT api/values/5 public void Put(int id, string value) { } // DELETE api/values/5 public void Delete(int id) { }} |
Key Http Support |
Note that the methods above - Get(), Post(), Put(), Delete(). These methods map to specific CRUD operations and HTTP verbs executed by the Windows 8 application. Because it is based on REST, any client capable of http can call into these methods. |
Automatic routing of web requests |
This is the magic of the ASP.NET Web API framework: it automatically routes the HTTP verbs used by the client directly to the methods defined in the VisualController class, minimizing potential programming mistakes. |
Our LocationWebService project is ready to run right now. |
The methods in the ValuesController class are self-documenting. Note that the Get() method in the code below gets called when the client issues the HTTP verb get using the following URL: https://127.0.0.1:81/api/values(The port differs from system to system. If we deploy to a data center, this port is not relevant. The port matters only if you run your project locally on your development machine)
|
1234 |
public IEnumerable<string> Get() { return new string[] { "value1", "value2" }; } | |
Note the video which shows us exactly how to call into a web service method
- Let us now use a browser to test our web service.
- Any browser can be used for this purpose.
- To test the Web Service from a browser, perform the following steps:
- In Visual Studio, click on the Debug menu and choose Start Debugging.
- This will start the various emulators to allow us to run our application on our local dev machine.
- There is a Compute Emulator
- There is a Storage Emulator
- We are not using storage today for this app
- You should see the screen above.
- Notice the web address of https://128.0.0.1:81
- That is port 81 on my machine (yours may differ)
- We can call the get() method by simply issuing the following url
- https://127.0.0.1:81/api/values
- This will trigger the ASP.NET Web API application to send the two strings:
- Watch the video that illustrates this application running and returning value1 and value2
- We just ran a simple intro sample before we do the real work.
- Now should starting to see that we can call into the cloud application quite easily. We just need to use a URL from a Windows 8 Application.
- We can pass parameters and receive data back.
- The data we pass will be GPS coordinates.
- The data we get back will be location information, such as neighborhood, city, etc.
- Conclusion
- We have successfully tested an MVC Web API based cloud project.
- The next step is to enhance it to call another web service to get location information based on GPS coordinates
- This is Step 3
- Question
- Should I keep doing quick videos? Are they helpful?
- No voice yet. Pretty self-explanatory what I'm doing.
|