Retrieve Calendar Information Using EWS Managed API 2.2 and PowerShell
Retrieve Calendar Information Using EWS Managed API 2.2 and PowerShell
Summary
This TechNet Wiki article is to demo a PowerShell script which retrieves calendar information of a given mailbox. For this we used EWS Managed API 2.2 in PowerShell.
References
- Download EWS Managed API
- How to: Communicate with EWS using EWS Managed API
- Get started with EWS Managed API client applications
- EWS Managed API reference
Solution
To achieve this requirement, we need to follow the steps:
# Load the assembly
Import-Module 'C:\Program Files\Microsoft\Exchange\Web Services\2.2\Microsoft.Exchange.WebServices.dll'
# Pass the credential
$Admin = "chendrayan@contoso.onmicrosoft.com"
$Password = "SuperSecretPassword" | ConvertTo-SecureString -AsPlainText -Force
# Instantiate the Exchange Service Class
$Service = [Microsoft.Exchange.WebServices.Data.ExchangeService]::new([Microsoft.Exchange.WebServices.Data.ExchangeVersion]::Exchange2013_SP1)
# Set the credentials properties
$Service.Credentials = [System.Net.NetworkCredential]::new($Admin,$Password)
# Define the URL property
$Service.Url = "https://outlook.office365.com/EWS/Exchange.asmx"
# Using the Bind method in CalendarFolder class with the overloads $Service and Folder ID or name
$Folder = [Microsoft.Exchange.WebServices.Data.CalendarFolder]::Bind($Service,[Microsoft.Exchange.WebServices.Data.WellKnownFolderName]::Calendar)
# Define the view using CalendarView class - In our case searching calendar information for next 3 days
$View = [Microsoft.Exchange.WebServices.Data.CalendarView]::new([datetime]::Now,[datetime]::Now.AddDays(3))
# Load only required properties using the property set
$View.PropertySet = [Microsoft.Exchange.WebServices.Data.PropertySet]::new([Microsoft.Exchange.WebServices.Data.AppointmentSchema]::Subject,
[Microsoft.Exchange.WebServices.Data.AppointmentSchema]::Start,[Microsoft.Exchange.WebServices.Data.AppointmentSchema]::End,
[Microsoft.Exchange.WebServices.Data.AppointmentSchema]::DateTimeSent)
# Using the Find appointments methods - Get the output
$Folder.FindAppointments($View) | Select-Object start , End , Subject
Output 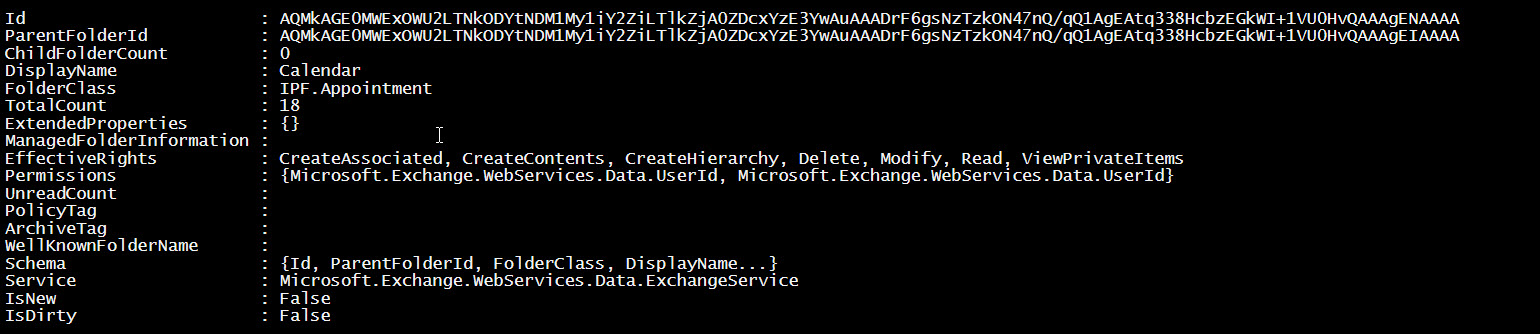
Full Code
function Get-xCalendarInformation
{
<#
.SYNOPSIS
A PowerShell function to list calendar information
.DESCRIPTION
Long description
.EXAMPLE
PS C:\> Get-xCalendarInformation -Identity "user@tenant.onmicrosoft.com" -days 3
List next 3 days calendar information
.NOTES
@ChendrayanV
http://chen.about-powershell.com
#>
[CmdletBinding()]
param (
[Parameter(Mandatory,ValueFromPipeline,ValueFromPipelineByPropertyName)]
$Identity,
[Parameter()]
$Days,
[Parameter()]
[System.Management.Automation.CredentialAttribute()]
[pscredential]
$Credential
)
begin
{
Import-Module 'C:\Program Files\Microsoft\Exchange\Web Services\2.2\Microsoft.Exchange.WebServices.dll'
}
process
{
$Service = [Microsoft.Exchange.WebServices.Data.ExchangeService]::new()
if($PSBoundParameters.ContainsKey('Credential'))
{
$Service.Credentials = [System.Net.NetworkCredential]::new($Credential.UserName,$Credential.Password)
}
else
{
$Service.UseDefaultCredentials = $true
}
$Service.Url = "https://outlook.office365.com/EWS/Exchange.asmx"
$Folder = [Microsoft.Exchange.WebServices.Data.CalendarFolder]::Bind($Service,[Microsoft.Exchange.WebServices.Data.WellKnownFolderName]::Calendar)
$View = [Microsoft.Exchange.WebServices.Data.CalendarView]::new([datetime]::Now,[datetime]::Now.AddDays($Days))
$View.PropertySet = [Microsoft.Exchange.WebServices.Data.PropertySet]::new([Microsoft.Exchange.WebServices.Data.AppointmentSchema]::Subject,
[Microsoft.Exchange.WebServices.Data.AppointmentSchema]::Start,[Microsoft.Exchange.WebServices.Data.AppointmentSchema]::End,
[Microsoft.Exchange.WebServices.Data.AppointmentSchema]::DateTimeSent)
$Folder.FindAppointments($View) | Select-Object start , End , Subject
}
end
{
}
}
Usage
help Get-xCalendarInformation -Examples