Hi all,
thank you so much your kindly replies, I'm in learning process of Blazor, and I use NET Core 9 (VS 2022).
Ok, I have created a new Blazor project (web app) + PosgreSQL-14
program.cs
using M2M.Components;
using M2M.Components.Account;
using M2M.Data;
using Microsoft.AspNetCore.Components.Authorization;
using Microsoft.AspNetCore.Identity;
using Microsoft.EntityFrameworkCore;
var builder = WebApplication.CreateBuilder(args);
string pgSQLServer = builder.Configuration.GetSection("PG_SQL").GetSection("Server").Value;
string pgSQLDBName = builder.Configuration.GetSection("PG_SQL").GetSection("DB_Name").Value;
string pgSQLDBUser = builder.Configuration.GetSection("PG_SQL").GetSection("DB_User").Value;
string pgSQLDBPassword = builder.Configuration.GetSection("PG_SQL").GetSection("DB_User_Password").Value;
int pgPoolSize = Convert.ToInt32(builder.Configuration.GetSection("PG_SQL").GetSection("DB_Pool_Size").Value);
string pgSQLConnectionString = "Server=" + M2M.Models.AppSettings.PG_SQL.Server + ";Database=" + M2M.Models.AppSettings.PG_SQL.DB_Name + ";Port=5432;User ID=" + M2M.Models.AppSettings.PG_SQL.DB_User + ";Password=" + M2M.Models.AppSettings.PG_SQL.DB_User_Password + ";Keepalive=300; Pooling = true; Minimum Pool Size = 0; Maximum Pool Size = " + pgPoolSize + "; Connection Idle Lifetime=300; CommandTimeout=300; Tcp Keepalive=true; Include Error Detail=true";
M2M.Models.AppSettings.PG_SQL.Connection_String = pgSQLConnectionString;
// Add services to the container.
builder.Services.AddRazorComponents()
.AddInteractiveServerComponents();
builder.Services.AddCascadingAuthenticationState();
builder.Services.AddScoped<IdentityUserAccessor>();
builder.Services.AddScoped<IdentityRedirectManager>();
builder.Services.AddScoped<AuthenticationStateProvider, IdentityRevalidatingAuthenticationStateProvider>();
builder.Services.AddAuthentication(options =>
{
options.DefaultScheme = IdentityConstants.ApplicationScheme;
options.DefaultSignInScheme = IdentityConstants.ExternalScheme;
})
.AddIdentityCookies();
builder.Services.AddDbContext<ApplicationDbContext>(options =>
options.UseNpgsql(pgSQLConnectionString));
builder.Services.AddDatabaseDeveloperPageExceptionFilter();
builder.Services.AddIdentityCore<ApplicationUser>(options => options.SignIn.RequireConfirmedAccount = true)
.AddEntityFrameworkStores<ApplicationDbContext>()
.AddSignInManager()
.AddDefaultTokenProviders();
builder.Services.AddSingleton<IEmailSender<ApplicationUser>, IdentityNoOpEmailSender>();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseMigrationsEndPoint();
}
else
{
app.UseExceptionHandler("/Error", createScopeForErrors: true);
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseAntiforgery();
app.MapStaticAssets();
app.MapRazorComponents<App>()
.AddInteractiveServerRenderMode();
// Add additional endpoints required by the Identity /Account Razor components.
app.MapAdditionalIdentityEndpoints();
app.Run();
type here
login.razor
@page "/"
@using System.ComponentModel.DataAnnotations
@using Microsoft.AspNetCore.Authentication
@using Microsoft.AspNetCore.Identity
@using M2M.Data
@inject SignInManager<ApplicationUser> SignInManager
@inject ILogger<Login> Logger
@inject NavigationManager NavigationManager
@inject IdentityRedirectManager RedirectManager
<PageTitle>Log in</PageTitle>
<h1>Log in</h1>
<div class="row">
<div class="col-lg-6">
<section>
<StatusMessage Message="@errorMessage" />
<EditForm Model="Input" method="post" OnValidSubmit="LoginUser" FormName="login">
<DataAnnotationsValidator />
<h2>Use a local account to log in.</h2>
<hr />
<ValidationSummary class="text-danger" role="alert" />
<div class="form-floating mb-3">
<InputText @bind-Value="Input.Email" id="Input.Email" class="form-control" autocomplete="username" aria-required="true" placeholder="name@example.com" />
<label for="Input.Email" class="form-label">Email</label>
<ValidationMessage For="() => Input.Email" class="text-danger" />
</div>
<div class="form-floating mb-3">
<InputText type="password" @bind-Value="Input.Password" id="Input.Password" class="form-control" autocomplete="current-password" aria-required="true" placeholder="password" />
<label for="Input.Password" class="form-label">Password</label>
<ValidationMessage For="() => Input.Password" class="text-danger" />
</div>
<div class="checkbox mb-3">
<label class="form-label">
<InputCheckbox @bind-Value="Input.RememberMe" class="darker-border-checkbox form-check-input" />
Remember me
</label>
</div>
<div>
<button type="submit" class="w-100 btn btn-lg btn-primary">Log in</button>
</div>
<div>
<p>
<a href="Account/ForgotPassword">Forgot your password?</a>
</p>
<p>
<a href="@(NavigationManager.GetUriWithQueryParameters("Account/Register", new Dictionary<string, object?> { ["ReturnUrl"] = ReturnUrl }))">Register as a new user</a>
</p>
<p>
<a href="Account/ResendEmailConfirmation">Resend email confirmation</a>
</p>
</div>
</EditForm>
</section>
</div>
<div class="col-lg-4 col-lg-offset-2">
<section>
<h3>Use another service to log in.</h3>
<hr />
<ExternalLoginPicker />
</section>
</div>
</div>
@code {
private string? errorMessage;
[CascadingParameter]
private HttpContext HttpContext { get; set; } = default!;
[SupplyParameterFromForm]
private InputModel Input { get; set; } = new();
[SupplyParameterFromQuery]
private string? ReturnUrl { get; set; }
protected override async Task OnInitializedAsync()
{
if (HttpMethods.IsGet(HttpContext.Request.Method))
{
// Clear the existing external cookie to ensure a clean login process
await HttpContext.SignOutAsync(IdentityConstants.ExternalScheme);
}
}
public async Task LoginUser()
{
// This doesn't count login failures towards account lockout
// To enable password failures to trigger account lockout, set lockoutOnFailure: true
var result = await SignInManager.PasswordSignInAsync(Input.Email, Input.Password, Input.RememberMe, lockoutOnFailure: false);
if (result.Succeeded)
{
Logger.LogInformation("User logged in.");
RedirectManager.RedirectTo(ReturnUrl);
}
else if (result.RequiresTwoFactor)
{
RedirectManager.RedirectTo(
"Account/LoginWith2fa",
new() { ["returnUrl"] = ReturnUrl, ["rememberMe"] = Input.RememberMe });
}
else if (result.IsLockedOut)
{
Logger.LogWarning("User account locked out.");
RedirectManager.RedirectTo("Account/Lockout");
}
else
{
errorMessage = "Error: Invalid login attempt.";
}
}
private sealed class InputModel
{
[Required]
[EmailAddress]
public string Email { get; set; } = "";
[Required]
[DataType(DataType.Password)]
public string Password { get; set; } = "";
[Display(Name = "Remember me?")]
public bool RememberMe { get; set; }
}
}
with the above codes (I don't know what to do), I just run it, but I got the following exception
System.ArgumentException: 'Host can't be null'
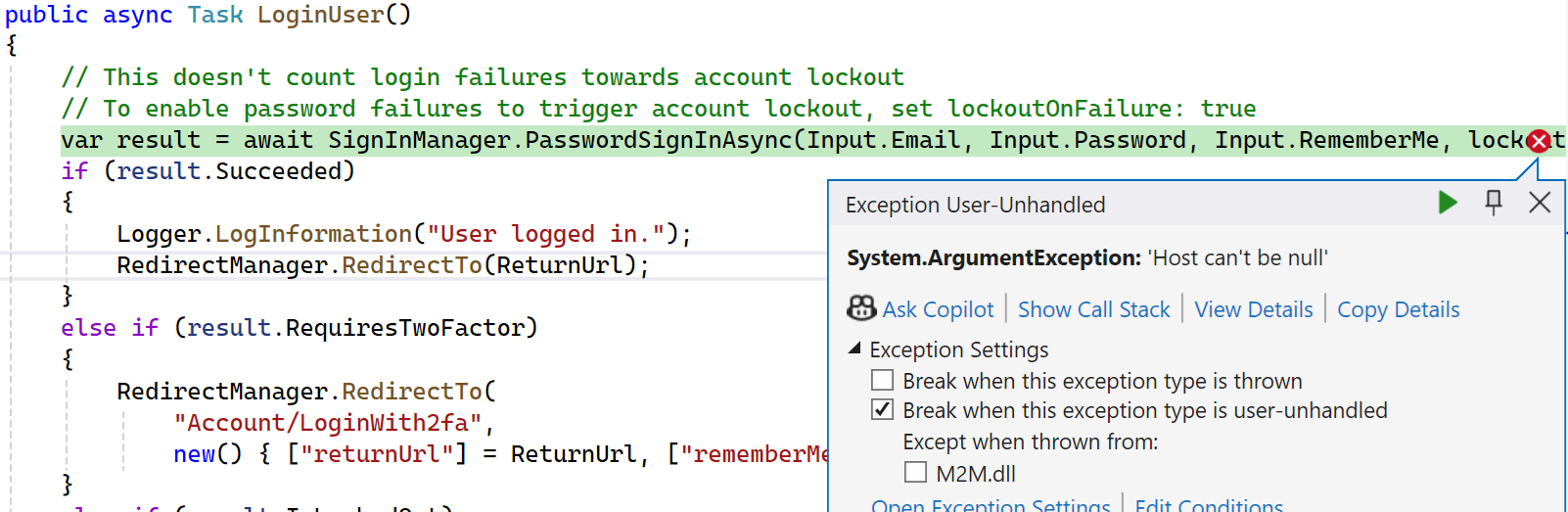
last but not least, I have my own User_Login.cs, and I want when the user clicks Login button, then it will executes the below, how to do this?
User_Login.cs
try
{
using (NpgsqlConnection conn = new NpgsqlConnection(Models.AppSettings.PG_SQL.Connection_String))
{
try
{
string xUserEmail = DES.TripleDesEncrypt(userEmail.Trim().ToLower());
string xUserPassword = DES.TripleDesEncrypt(userPassword.Trim());
string sql = "select u.pid usersPid, u.user_name userName, u.user_password, u.user_email userEmail, u.user_mobile userMobile, u.user_role userRole, u.super super, u.last_login " +
"From users u " +
"u.user_password=@User_Password And " +
"u.active=true";
using (var dr = conn.ExecuteReader(sql, new
{
User_Email = xUserEmail,
User_Password = xUserPassword
}))
{
if (dr.Read())
{
// do some process here
}
}
}
catch (Exception e)
{
// blah blah
}
finally { }
}