Hello. I have a logic app that needs specific roles assigned to it in order to function properly, so I have been using the Microsoft.Graph cmdlet New-MgServicePrincipalAppRoleAssignedTo to assign those roles.
First, I have my powershell script assign some Windows Defender ATP roles to my logic app, and this works perfectly fine. here is a snippet of that code
$AppId = "fc780465-2017-40d4-a0c5-307022471b92"
# Attempt to retrieve the service principal for the Logic App
$LogicApp = Get-MgServicePrincipal -Filter "displayName eq '$LogicAppName'"
if (-not $LogicApp) {
Write-Output "Logic app `"$LogicAppName`" not found."
break
}
# Attempt to retrieve the service principal for the specified AppId
$oWDATPSpn = Get-MgServicePrincipal -Filter "appId eq '$AppId'"
# Loop through each permission and attempt to assign it
foreach ($perm in $perms) {
$AppRoles = $oWDATPSpn.AppRoles | Where-Object { $_.Value -eq $perm -and $_.AllowedMemberTypes -contains "Application" }
foreach ($AppRole in $AppRoles) {
try {
Write-Output "Assigning $($AppRole.Value) to $LogicAppName"
$assignment = New-MgServicePrincipalAppRoleAssignedTo -ServicePrincipalId $oWDATPSpn.Id -AppRoleId $AppRole.Id -PrincipalId $LogicApp.Id -ResourceId $oWDATPSpn.Id
} catch {
Write-Output $_.Exception.Message
}
}
}
Here is the issue, when I try to do the same thing for Microsoft Graph permissions, I get an error "Insufficient privileges to complete the operation."
$perms2 = @(
"IdentityRiskyUser.ReadWrite.All",
"User.Read.All"
)
$AppId2 = "00000003-0000-0000-c000-000000000000"
$MGSpn = Get-MgServicePrincipal -Filter "appId eq '$AppId2'"
foreach ($perm2 in $perms2) {
$AppRoles2 = $MGSpn.AppRoles | Where-Object { $_.Value -eq $perm2 -and $_.AllowedMemberTypes -contains "Application" }
foreach ($AppRole2 in $AppRoles2) {
try {
Write-Output "Assigning $($AppRole2.Value) to $LogicAppName"
$assignment = New-MgServicePrincipalAppRoleAssignedTo -ServicePrincipalId $MGSpn.Id -AppRoleId $AppRole2.Id -PrincipalId $LogicApp.Id -ResourceId $MGSpn.Id
} catch {
Write-Output $_.Exception.Message
}
}
}
Here is the specific error
Does anyone know why I would be getting this error for Microsoft Graph roles and not Windows Defender ATP roles?
Before running the script, I did run "Connect-MgGraph -Scopes "Application.ReadWrite.All", "Directory.ReadWrite.All"" and the account I am running it through has global administrator and is an owner of the subscription. I am also added as a user under Microsoft Graph Powershell. I also know the code works because I have tried the same exact same script on a different account and subscription, and it worked with no errors.
Here is the full list of my current roles
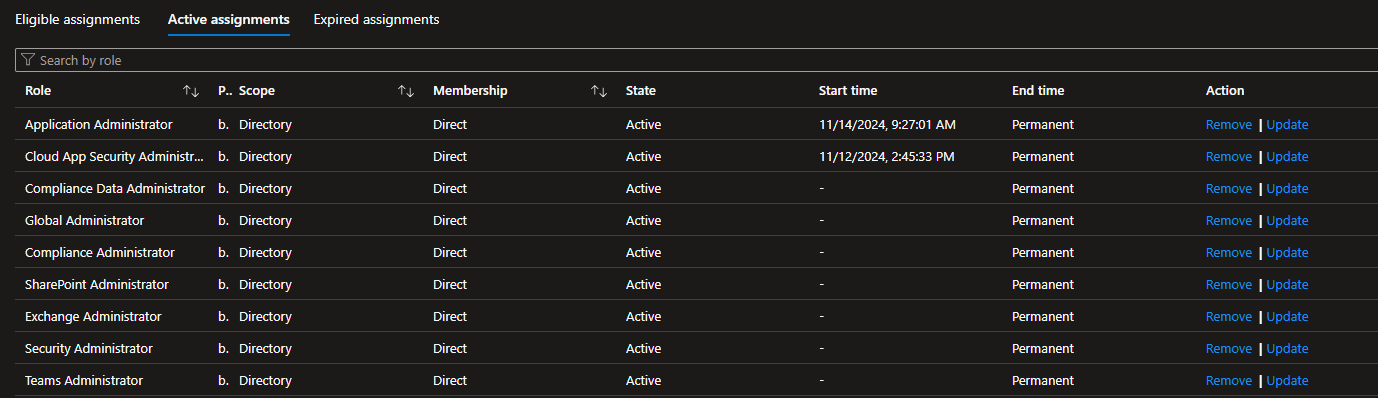
I do not know what permissions I am missing on this account, or how my authorization is being denied specifically for graph permissions, so help would be much appreciated. Thanks!