Hi @Falanga, Rod, DOH
I do believe that I have a problem if I tried to introduce an identity property to one of the columns. For example, the primary key column on most of the tables have some missing values. There's values like 1, 3, 4, 5, 7, 8, 11, etc. When defining an identity column, whether it is a primary key or not, you can specify a seed and an interval. There's no way I can specify an interval that allows for +1 in some cases, then +2 or +3, etc. in other cases. And if that table is the primary key, then the foreign key relationship gets totally messed up if I can't match the values in the primary key to the foreign key values in the related table.
Based on the table (timetrack
and r_job
) definition, do you mean the jobid
column in timetrack might contain value not in the jobid
column in r_job
table? If that is the case, when you set the foreign key, it will cause the foreign key constraint error.
So before setting the foreign key, you can query to check the records in timetrack
that are causing the conflict, and then handle them:
SELECT *
FROM timetrack
WHERE jobid NOT IN (SELECT jobid FROM r_job);
Then, you can refer to the following steps to configure foreign key using EF core methods:
1.In the Asp.net core application appsettings.json file set the database connection string like this:
{
"ConnectionStrings": {
"DefaultConnection": "your database connection string"
},
2.Use Scaffolding (Reverse Engineering) to generate classes based on the existing database and tables (check the Scaffolding Prerequisites and require install EF Core Tools).
In my MVC application, right click the project and select the Open in Terminal option, in the terminal console executes the following command to generate the class:
dotnet ef dbcontext scaffold "Name=ConnectionStrings:DefaultConnection" Microsoft.EntityFrameworkCore.SqlServer --output-dir Models --context-dir Data --context ApplicationDbContext --force --table r_job --table timetrack
the output as below: it will generate the RJob and Timetrack class and add the relate DbSet in the ApplicationDbContext:
3.Then in the RJob, Timetrack and ApplicationDbContext class, you can add the navigation property and configure relationship and add the foreign key:
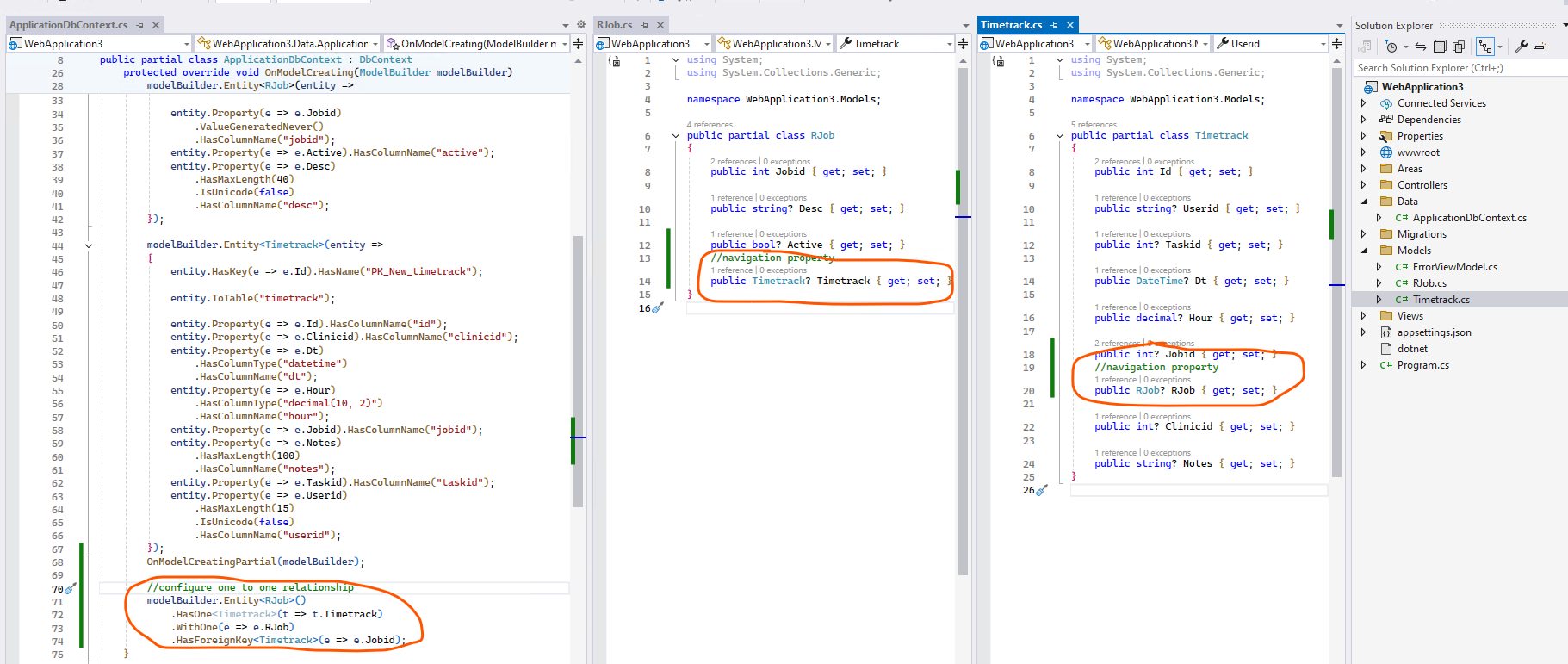
4.In the Package Manger Console, run the add-migration addonetooneforeignkey
command, in the migration file, change the content as below:
public partial class addonetooneforeignkey : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.AlterColumn<int>(
name: "jobid",
table: "timetrack",
type: "int",
nullable: true,
oldClrType: typeof(int),
oldType: "int");
migrationBuilder.CreateIndex(
name: "IX_timetrack_jobid",
table: "timetrack",
column: "jobid",
unique: true,
filter: "[jobid] IS NOT NULL");
migrationBuilder.AddForeignKey(
name: "FK_timetrack_r_job_jobid",
table: "timetrack",
column: "jobid",
principalTable: "r_job",
principalColumn: "jobid");
}
/// <inheritdoc />
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropForeignKey(
name: "FK_timetrack_r_job_jobid",
table: "timetrack");
migrationBuilder.DropIndex(
name: "IX_timetrack_jobid",
table: "timetrack");
migrationBuilder.AlterColumn<int>(
name: "jobid",
table: "timetrack",
type: "int",
nullable: false,
defaultValue: 0,
oldClrType: typeof(int),
oldType: "int",
oldNullable: true);
}
}
5.After that, running the update-database
command in the Package Manger Console to update the database.
6.After refresh the database from SSMS, you can see jobid column in the timetrack already is the foreign key.
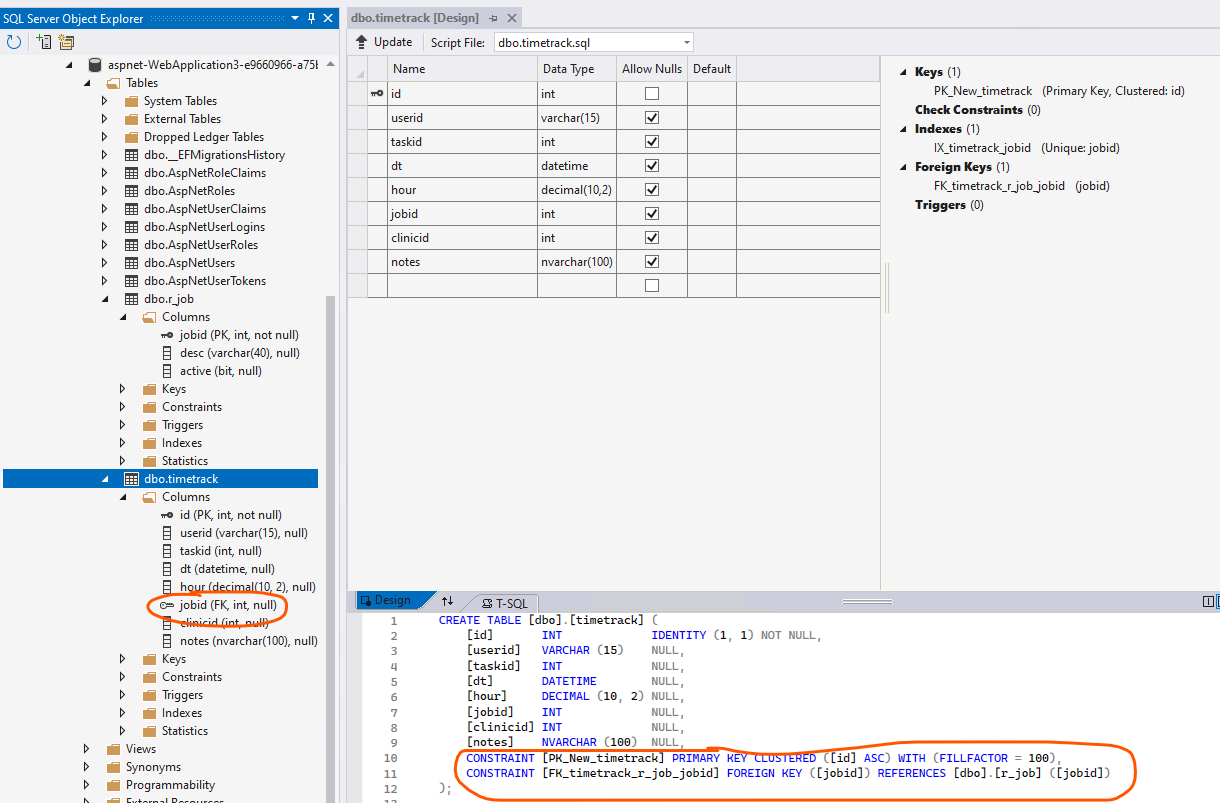
In my above sample, I configure them with One-to-one relationships, you can also configure other relationships for them, refer to Introduction to relationships.
Besides, you can also try to use sql command to add the foreign key and then use the Scaffolding (Reverse Engineering) to generate classes based on the existing database.
Refer to #SQL FOREIGN KEY on ALTER TABLE
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.
Best regards,
Dillion