Hi Gino,
I can share PowerShell example with you. https://github.com/iFestral/akdotms/blob/main/EntraID-List-AllUsersGroups.ps1
I'm filling a List with Users and their groups.
Setting of the column:
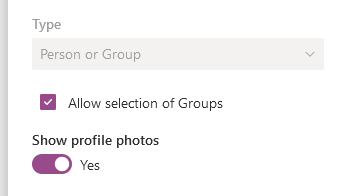
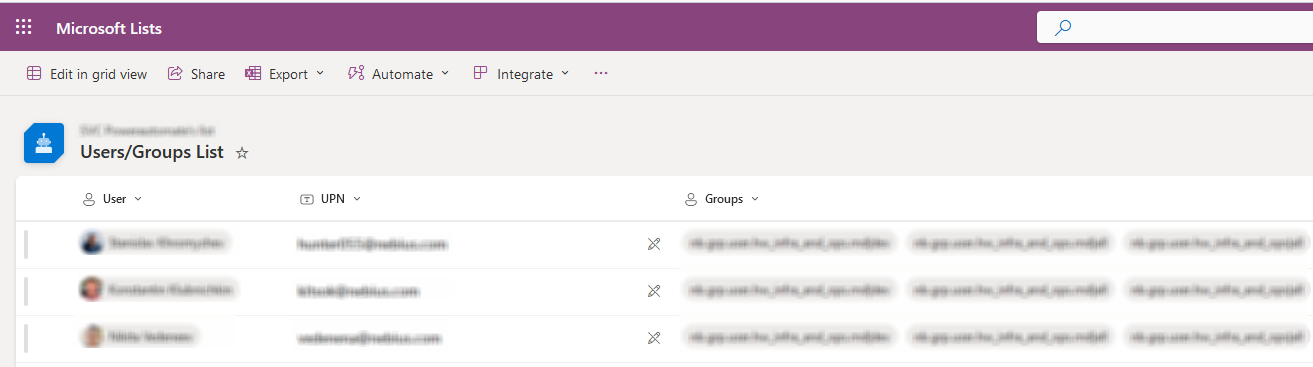
Python example:
import msal
import requests
# Authentication to Microsoft Graph API
tenant_id = 'YOUR_TENANT_ID'
client_id = 'YOUR_CLIENT_ID'
client_secret = 'YOUR_CLIENT_SECRET'
# Set up authentication for Microsoft Graph
authority = f"https://login.microsoftonline.com/{tenant_id}"
scopes = ["https://graph.microsoft.com/.default"]
app = msal.ConfidentialClientApplication(
client_id, authority=authority, client_credential=client_secret
)
# Get access token for Graph API and SharePoint
token_result = app.acquire_token_for_client(scopes=scopes)
if "access_token" in token_result:
access_token = token_result['access_token']
# Microsoft Graph and SharePoint setup
sharepoint_list_name = "YOUR_SHAREPOINT_LIST_NAME"
users = [
# List of users, you can replace with actual user objects
{"Id": "USER_ID_1", "UserPrincipalName": "user1@domain.com"},
{"Id": "USER_ID_2", "UserPrincipalName": "user2@domain.com"}
]
sharepoint_site_url = f"https://your_sharepoint_site_url/_api/web/lists/getbytitle('{sharepoint_list_name}')/items"
# Get List of items from SharePoint
list_items_response = requests.get(
sharepoint_site_url,
headers={"Authorization": f"Bearer {access_token}", "Accept": "application/json;odata=verbose"}
)
if list_items_response.status_code == 200:
list_items = list_items_response.json()['d']['results']
for user in users:
# Check if the user is already in the SharePoint list
if not any(item['Title'] == user['UserPrincipalName'] for item in list_items):
try:
# Get user groups from Microsoft Graph
graph_url = f"https://graph.microsoft.com/v1.0/users/{user['Id']}/memberOf"
graph_headers = {'Authorization': 'Bearer ' + access_token}
groups_response = requests.get(graph_url, headers=graph_headers)
if groups_response.status_code == 200:
groups = groups_response.json()['value']
filtered_groups = [
g['displayName'] for g in groups if 'akdotms.grp.user.' in g['displayName']
]
# Create new item in SharePoint
new_item_data = {
"Title": user['UserPrincipalName'],
"User": user['UserPrincipalName'],
"Groups": ', '.join(filtered_groups)
}
create_item_response = requests.post(
sharepoint_site_url,
headers={"Authorization": f"Bearer {access_token}", "Content-Type": "application/json"},
json=new_item_data
)
if create_item_response.status_code == 201:
print(f"Created item for {user['UserPrincipalName']}")
else:
print(f"Failed to create item: {create_item_response.status_code} - {create_item_response.text}")
except Exception as e:
print(f"Failed to create List item for {user['UserPrincipalName']}: {str(e)}")
else:
try:
# Get the SharePoint item to update
item_to_update = next((item for item in list_items if item['Title'] == user['UserPrincipalName']), None)
if item_to_update:
# Get user groups from Microsoft Graph
graph_url = f"https://graph.microsoft.com/v1.0/users/{user['Id']}/memberOf"
graph_headers = {'Authorization': 'Bearer ' + access_token}
groups_response = requests.get(graph_url, headers=graph_headers)
if groups_response.status_code == 200:
groups = groups_response.json()['value']
filtered_groups = [
g['displayName'] for g in groups if 'akdotms.grp.user.' in g['displayName']
]
# Update existing item in SharePoint
update_item_data = {
"Groups": ', '.join(filtered_groups)
}
update_item_url = f"{sharepoint_site_url}({item_to_update['Id']})"
update_item_response = requests.post(
update_item_url,
headers={"Authorization": f"Bearer {access_token}", "Content-Type": "application/json"},
json=update_item_data
)
if update_item_response.status_code == 204:
print(f"Updated item for {user['UserPrincipalName']}")
else:
print(f"Failed to update item: {update_item_response.status_code} - {update_item_response.text}")
except Exception as e:
print(f"Failed to update List item for {user['UserPrincipalName']}: {str(e)}")
else:
print(f"Failed to retrieve SharePoint list items: {list_items_response.status_code} - {list_items_response.text}")
else:
print(f"Error retrieving access token: {token_result.get('error_description')}")
Best regards,
Aleksandr
If the response is helpful, please click "Accept Answer" and upvote it.