Hi, @mc
Use ellipse
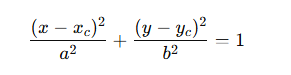
and line.
point 1
and point c
are known points. In question, it is x1,y1
x,y
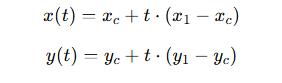
So:
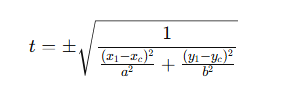
#include <iostream>
#include <cmath>
#include <vector>
struct Point {
double x, y;
};
Point findIntersection(double ellipseX, double ellipseY, double MajorAxis, double MinorAxis, double x1, double y1) {
std::vector<Point> intersections;
// cal delta_x , delta_y
double dx = x1 - ellipseX;
double dy = y1 - ellipseY;
double A = (dx * dx) / (MajorAxis * MajorAxis) + (dy * dy) / (MinorAxis * MinorAxis);
if (A <= 1) {
std::cout << "the point is inside or on an ellipse\n";
return Point(x1, y1);
}
double t1 = std::sqrt(1.0 / A);
double t2 = -t1;
//two intersections
intersections.push_back({ ellipseX + t1 * dx, ellipseY + t1 * dy });
intersections.push_back({ ellipseX + t2 * dx, ellipseY + t2 * dy });
for (const auto& intersection : intersections) {
if ((intersection.x - x1) * (ellipseX - intersection.x) >= 0 &&
(intersection.y - y1) * (ellipseY - intersection.y) >= 0) {
std::cout << "Intersection: (" << intersection.x << ", " << intersection.y << ")\n";
return intersection;
}
}
}
int main() {
double ellipseX = 0.0, ellipseY = 0.0; // center
double MajorAxis = 5.0, MinorAxis = 3.0;
double pX = 6.0, pY = 0.0; // point
Point intersection = findIntersection(ellipseX, ellipseY, MajorAxis, MinorAxis, pX, pY);
return 0;
}
Best regards,
Minxin Yu
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.