I am using CommunityToolkit MVVM to add and delete records. However, when I add records and navigate to another page as a popup, the command does not trigger. I have attached the project and a screenshot for your reference.
<CollectionView ItemsSource="{Binding Employees,Mode=TwoWay}"
BackgroundColor="Gray"
HorizontalScrollBarVisibility="Never"
VerticalScrollBarVisibility="Never"
VerticalOptions="FillAndExpand"
HorizontalOptions="FillAndExpand"
ItemsUpdatingScrollMode="KeepItemsInView"
ItemSizingStrategy="MeasureAllItems"
Margin="5,100,5,10">
<CollectionView.ItemsLayout>
<GridItemsLayout Orientation="Vertical" VerticalItemSpacing="5"/>
</CollectionView.ItemsLayout>
<CollectionView.ItemTemplate>
<DataTemplate x:DataType="{x:Type models:EmployeeModel}">
<Border BackgroundColor="White"
StrokeShape="RoundRectangle 10"
Stroke="Black"
StrokeThickness="1"
Padding="0"
Margin="0">
<Grid ColumnDefinitions="*,*,*"
Padding="10,5"
HorizontalOptions="FillAndExpand"
VerticalOptions="FillAndExpand">
<Label Text="{Binding FirstName}"
Grid.Column="0"
TextColor="Black"
FontFamily="OpenSansRegular"
FontSize="16"/>
<Label Text="{Binding LastName}"
Grid.Column="1"
TextColor="Black"
FontFamily="OpenSansRegular"
FontSize="16"/>
<Button Text="Delete"
Grid.Column="2"
Command="{Binding Source={RelativeSource AncestorType={x:Type viewModels:EmployeeViewModel}}, Path=DeleteEmployeeCommand}"
CommandParameter="{Binding .}">
</Button>
</Grid>
<Border.GestureRecognizers>
<TapGestureRecognizer NumberOfTapsRequired="1"
CommandParameter="{Binding .}"
Command="{Binding Source={RelativeSource AncestorType={x:Type viewModels:EmployeeViewModel}}, Path=ViewEmployeeCommand}"/>
</Border.GestureRecognizers>
</Border>
</DataTemplate>
</CollectionView.ItemTemplate>
</CollectionView>
using System.Collections.ObjectModel;
namespace Maui_9.ViewModels
{
public partial class EmployeeViewModel : ObservableObject
{
/// <summary>
/// firstName
/// </summary>
[ObservableProperty]
private string firstName = string.Empty;
/// <summary>
/// firstName
/// </summary>
[ObservableProperty]
private string lastName = string.Empty;
/// <summary>
/// employees
/// </summary>
[ObservableProperty]
private ObservableCollection<EmployeeModel> employees = new();
/// <summary>
/// EmployeeViewModel
/// </summary>
/// <param name="employeeModels"></param>
public EmployeeViewModel(ObservableCollection<EmployeeModel>? employeeModels = null)
{
Employees.Clear();
if (employeeModels != null)
Employees = new ObservableCollection<EmployeeModel>(employeeModels);
}
/// <summary>
/// AddEmployee
/// </summary>
[RelayCommand]
private async Task AddEmployee()
{
EmployeeModel model = new EmployeeModel { FirstName = FirstName, LastName = LastName };
Employees.Add(model);
await MopupService.Instance.PushAsync(new EmployeeList(Employees), true);
}
/// <summary>
/// AddEmployee
/// </summary>
[RelayCommand]
private async Task DeleteEmployee(EmployeeModel employeeModel)
{
Employees.Remove(employeeModel);
await MopupService.Instance.PopAsync();
}
/// <summary>
/// AddEmployee
/// </summary>
[RelayCommand]
private async Task ViewEmployee(EmployeeModel employeeModel)
{
var page = Application.Current?.Windows[0].Page;
if (page != null)
await page.DisplayAlert("Employee", $"Viewing {employeeModel.FirstName} {employeeModel.LastName}", "OK");
}
}
}
namespace Maui_9.Pages;
public partial class EmployeeList : PopupPage
{
public EmployeeList()
{
InitializeComponent();
this.BindingContext = new EmployeeViewModel();
}
public EmployeeList(ObservableCollection<EmployeeModel> employeeModel)
{
InitializeComponent();
this.BindingContext = new EmployeeViewModel(employeeModel);
}
}
namespace Maui_9.Pages;
public partial class AddEmployee : ContentPage
{
public AddEmployee()
{
InitializeComponent();
this.BindingContext = new EmployeeViewModel();
}
}
1>E:\Practice_Projects\Maui_9\Pages\EmployeeList.xaml(54,47): XamlC warning XC0045: Binding: Property "ViewEmployeeCommand" not found on "Maui_9.Models.EmployeeModel".
1>E:\Practice_Projects\Maui_9\Pages\EmployeeList.xaml(47,33): XamlC warning XC0045: Binding: Property "DeleteEmployeeCommand" not found on "Maui_9.Models.EmployeeModel".
1>E:\Practice_Projects\Maui_9\Pages\EmployeeList.xaml(54,47): XamlC warning XC0045: Binding: Property "ViewEmployeeCommand" not found on "Maui_9.Models.EmployeeModel".
1>E:\Practice_Projects\Maui_9\Pages\EmployeeList.xaml(47,33): XamlC warning XC0045: Binding: Property "DeleteEmployeeCommand" not found on "Maui_9.Models.EmployeeModel".
I am encountering a "warning not found" issue, and the methods DeleteEmployeeCommand
and ViewEmployeeCommand
are not being triggered. However, if I remove the code x:DataType="{x:Type models:EmployeeModel}"
, the methods are called, but a null value is passed as the command parameter. Additionally, the screen displays blank data.
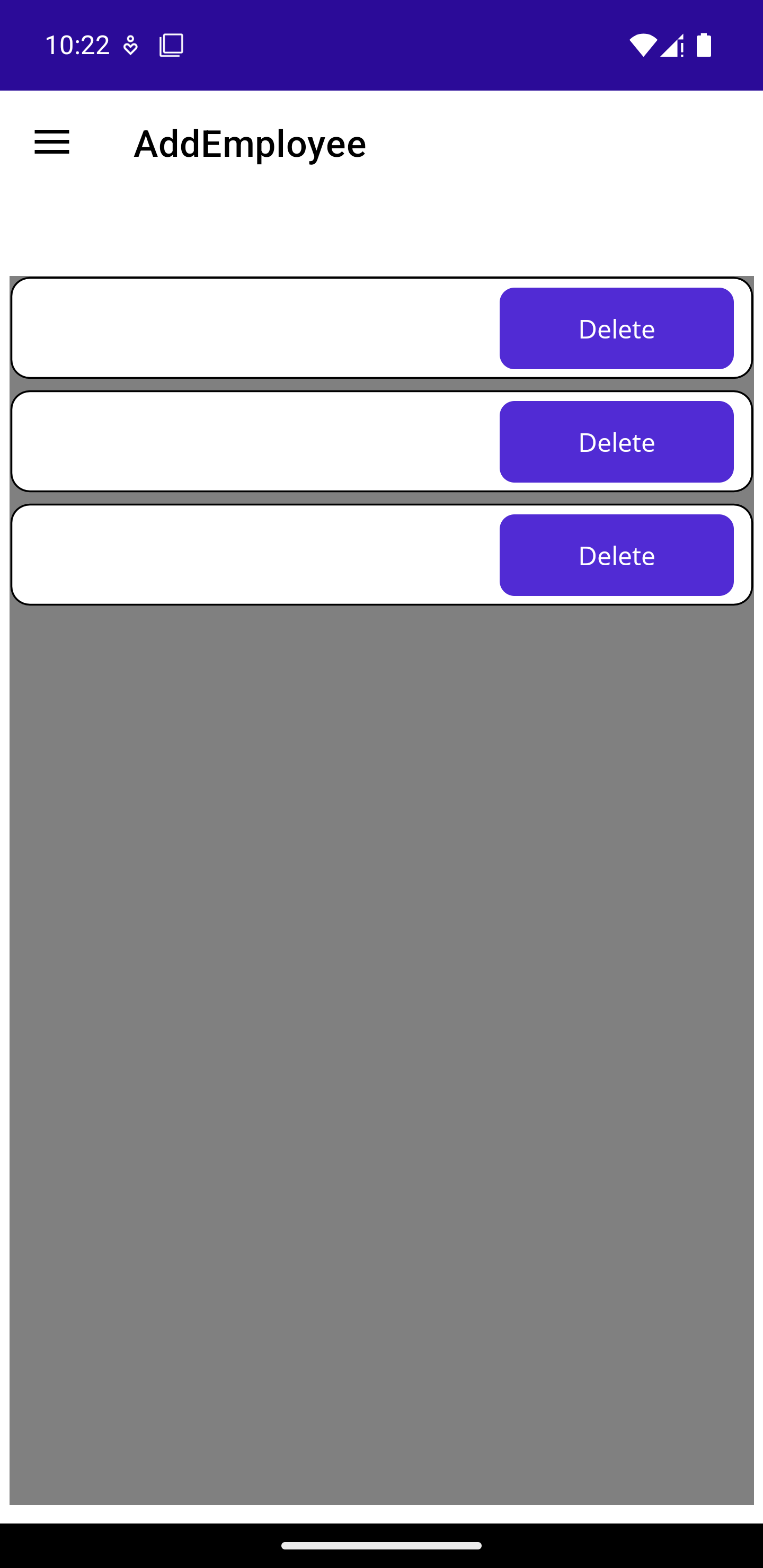