CORS is controlled by the site your blazor app is calling, not the blazor app/host itself. If you control the called site just add your GitHub page origin to the allowed list. if you don't control the site, then your only option is to create a new website that proxies the requests. On this new website you would enable CORS for your blazor app.
how to ALLOW CORS withing my blazor webassembly application
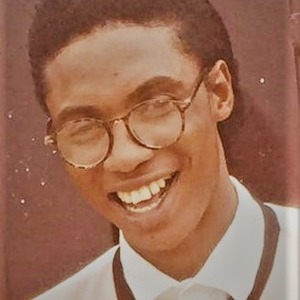
KwebenaAcquah-9104
306
Reputation points
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<!--<base href="https://www.capitalprints.com/" />-->
<title>CapitalPrint</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css">
<link rel="stylesheet" href="css/MainLayout.css">
<link rel="stylesheet" href="css/Home.css">
<link rel="stylesheet" href="css/all-products.css">
<link rel="stylesheet" href="css/Solution.css">
<link rel="stylesheet" href="css/Design.css">
<link rel="stylesheet" href="css/app.css">
<link rel="manifest" href="manifest.webmanifest"/>
<link rel="apple-touch-icon" sizes="512x512" href="icon-512.png" />
<link rel="apple-touch-icon" sizes="192x192" href="icon-192.png" />
<style>
.spinner {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
background-color: white;
z-index: 9999;
overflow: hidden;
}
.spinner-inner {
width: 100px;
height: 100px;
border: 10px solid #f3f3f3;
border-top: 10px solid mediumvioletred;
border-radius: 50%;
animation: spin 2s linear infinite;
position: relative;
}
.spinner-inner img {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 40px;
height: 40px;
}
@keyframes spin {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
</style>
</head>
<body>
<div id="app">
<div class="spinner">
<div class="spinner-inner">
<img src="favicon.ico" alt="CapitalPrint Logo">
</div>
</div>
</div>
<script src="_framework/blazor.webassembly.js"></script>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/js/bootstrap.bundle.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2canvas/1.4.1/html2canvas.min.js"></script>
<script>
async function captureAndSendImage(elementId, customText, selectedColor, price) {
try {
console.log("captureAndSendImage called:", elementId, customText, selectedColor, price);
const element = document.getElementById(elementId);
if (!element) {
console.error(`Element with ID '${elementId}' not found.`);
return;
}
// Capture the element as a canvas.
const canvas = await html2canvas(element);
const imageDataUrl = canvas.toDataURL("image/png");
console.log("Canvas captured. Converting to PNG.");
// Remove the "data:image/png;base64," prefix.
const base64ImageData = imageDataUrl.split(',')[1];
const formData = new FormData();
formData.append("image", base64ImageData);
// Post the image to imgbb. Replace the API key with your valid key.
const uploadResponse = await fetch('https://api.imgbb.com/1/upload?key=cc1582a60e5d59c570b5bfc6f6d4a6a7', {
method: 'POST',
body: formData
});
const data = await uploadResponse.json();
console.log("Image uploaded. Response received:", data);
// Verify that the upload returned a valid URL.
const uploadedImageUrl = data?.data?.url;
if (!uploadedImageUrl) {
throw new Error("Failed to retrieve uploaded image URL.");
}
// Create a message including the uploaded image URL directly.
const message = `Check out this product:\nName: ${customText}\nColor: ${selectedColor}\nPrice: GHS ${price}\nImage: ${uploadedImageUrl}`;
const phoneNumber = "233244736472";
const isMobile = /iPhone|iPad|iPod|Android/i.test(navigator.userAgent);
const whatsappUrl = isMobile
? `whatsapp://send?phone=${phoneNumber}&text=${encodeURIComponent(message)}`
: `https://wa.me/${phoneNumber}?text=${encodeURIComponent(message)}`;
console.log("Redirecting to:", whatsappUrl);
window.location.href = whatsappUrl;
} catch (error) {
console.error('Error capturing image or uploading:', error);
}
}
// ... existing drag functions remain unchanged ...
let dragElement = null;
let offsetX = 0;
let offsetY = 0;
function startDrag(event) {
dragElement = event.target;
offsetX = event.clientX - dragElement.getBoundingClientRect().left;
offsetY = event.clientY - dragElement.getBoundingClientRect().top;
document.addEventListener('mousemove', drag);
document.addEventListener('mouseup', stopDrag);
}
function drag(event) {
if (dragElement) {
dragElement.style.position = 'absolute';
dragElement.style.left = (event.clientX - offsetX) + 'px';
dragElement.style.top = (event.clientY - offsetY) + 'px';
}
}
function stopDrag() {
document.removeEventListener('mousemove', drag);
document.removeEventListener('mouseup', stopDrag);
dragElement = null;
}
</script>
<script>
function startChangingText(elementId, newText) {
const element = document.getElementById(elementId);
if (element) {
element.innerText = newText;
} else {
console.error(`Element with ID "${elementId}" not found.`);
}
}
</script>
<script>
function hideLoadingSpinner() {
const spinner = document.querySelector('.spinner');
if (spinner) {
spinner.style.display = 'none';
} else {
console.error('Spinner element not found.');
}
}
function startCarousel() {
$('#carouselExampleIndicators').carousel();
}
document.addEventListener('DOMContentLoaded', () => {
hideLoadingSpinner();
startCarousel();
});
</script>
</body>
</html>
i want to be able to deploy my application to github pages but this cors thing ... please I need help to fix this and deploy my webassembly application