i created a custom button control.
the class code is inherited from a button.
the problem is that in the class code i set the text to "REC" but when i drag the custom button control in form1 designer from the toolbox the default text is custombutton1.
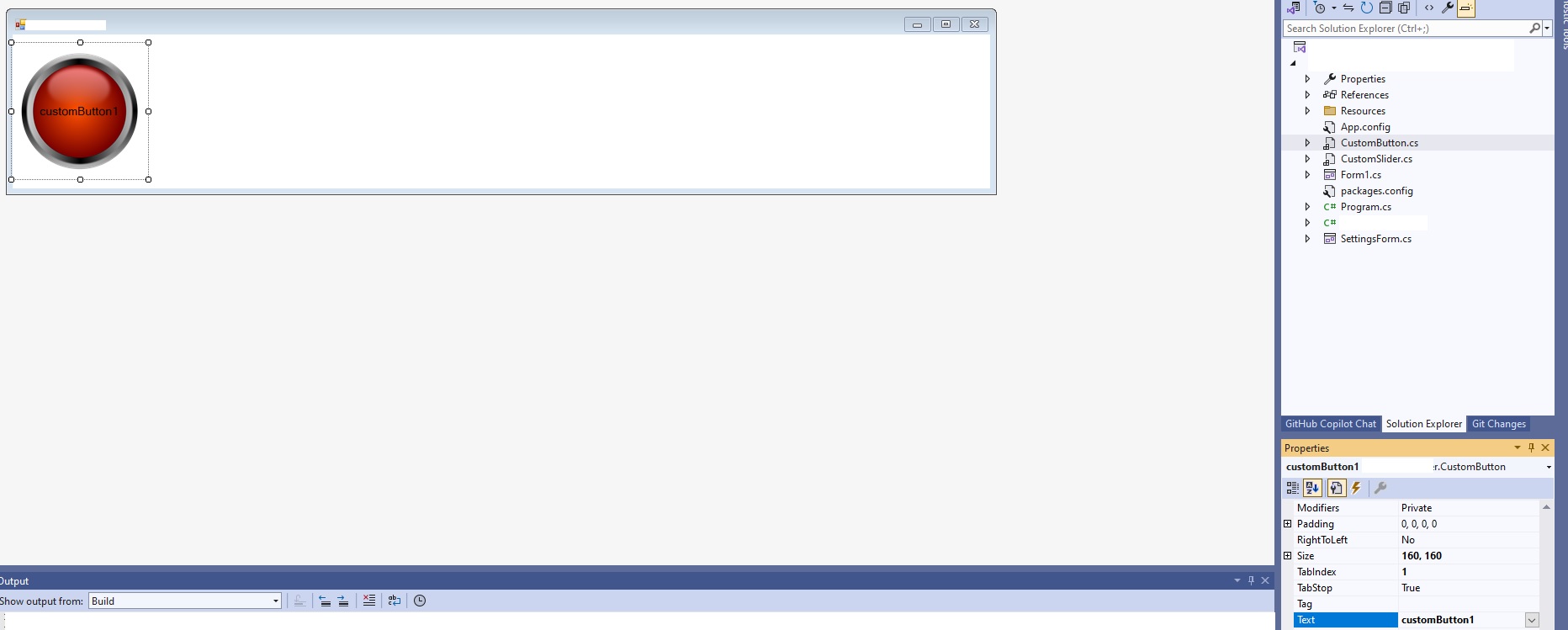
i want to make that when i drag the button from the toolbox the default text will be "REC".
using System;
using System.ComponentModel;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Drawing.Imaging;
using System.Windows.Forms;
namespace CB
{
public enum ButtonAlignment
{
Center,
Left,
Right,
TopLeft,
TopRight,
BottomLeft,
BottomRight
}
public class CustomButton : Button
{
private bool _DisplayFocusCues = true;
private Image _originalImage;
private Image _clickImage;
private Size _lastImageSize;
[Category("Layout")]
[Description("Sets the initial alignment of the button within its parent container.")]
public ButtonAlignment InitialAlignment { get; set; } = ButtonAlignment.Left;
public CustomButton()
{
this.FlatStyle = FlatStyle.Flat;
this.FlatAppearance.BorderSize = 0;
this.FlatAppearance.BorderColor = Color.FromArgb(0, 255, 255, 255);
this.FlatAppearance.MouseDownBackColor = Color.FromArgb(0, 255, 255, 255);
this.FlatAppearance.MouseOverBackColor = Color.FromArgb(0, 255, 255, 255);
this.DisplayFocusCues = false;
_originalImage = ResizeImage(Properties.Resources.pngimg_com___buttons_PNG152, 160,160);
this.Size = new Size(160, 160);
this.Image = _originalImage;
this.Text = "REC";
_clickImage = CreateClickImage(_originalImage);
_lastImageSize = _originalImage.Size;
}
protected override void OnParentChanged(EventArgs e)
{
base.OnParentChanged(e);
AlignButton(); // Align button when added to a parent container
}
private void AlignButton()
{
if (this.Parent == null) return;
int parentWidth = this.Parent.ClientSize.Width;
int parentHeight = this.Parent.ClientSize.Height;
switch (InitialAlignment)
{
case ButtonAlignment.Center:
this.Left = (parentWidth - this.Width) / 2;
this.Top = (parentHeight - this.Height) / 2;
break;
case ButtonAlignment.Left:
this.Left = 0;
this.Top = (parentHeight - this.Height) / 2;
break;
case ButtonAlignment.Right:
this.Left = parentWidth - this.Width;
this.Top = (parentHeight - this.Height) / 2;
break;
case ButtonAlignment.TopLeft:
this.Left = 0;
this.Top = 0;
break;
case ButtonAlignment.TopRight:
this.Left = parentWidth - this.Width;
this.Top = 0;
break;
case ButtonAlignment.BottomLeft:
this.Left = 0;
this.Top = parentHeight - this.Height;
break;
case ButtonAlignment.BottomRight:
this.Left = parentWidth - this.Width;
this.Top = parentHeight - this.Height;
break;
}
}
protected override void OnResize(EventArgs e)
{
base.OnResize(e);
this.Image = ResizeImage(_originalImage, this.Width, this.Height);
_clickImage = CreateClickImage(this.Image);
}
protected override bool ShowFocusCues => _DisplayFocusCues;
public bool DisplayFocusCues
{
get { return _DisplayFocusCues; }
set { _DisplayFocusCues = value; }
}
public new Image Image
{
get { return base.Image; }
set { base.Image = value; }
}
protected override void OnMouseDown(MouseEventArgs mevent)
{
base.OnMouseDown(mevent);
if (_clickImage != null)
this.Image = _clickImage;
}
protected override void OnMouseUp(MouseEventArgs mevent)
{
base.OnMouseUp(mevent);
this.Image = ResizeImage(_originalImage, this.Width, this.Height);
}
private Image ResizeImage(Image originalImage, int width, int height)
{
if (originalImage == null)
return null;
Bitmap resizedImage = new Bitmap(width, height);
using (Graphics gfx = Graphics.FromImage(resizedImage))
{
gfx.SmoothingMode = SmoothingMode.HighQuality;
gfx.InterpolationMode = InterpolationMode.HighQualityBicubic;
gfx.PixelOffsetMode = PixelOffsetMode.HighQuality;
gfx.DrawImage(originalImage, new Rectangle(0, 0, width, height));
}
return resizedImage;
}
private Image CreateClickImage(Image original)
{
if (original == null)
return null;
Bitmap clickedImage = new Bitmap(original);
using (Graphics g = Graphics.FromImage(clickedImage))
{
float brightness = 0.8f;
float[][] ptsArray =
{
new float[] {brightness, 0, 0, 0, 0},
new float[] {0, brightness, 0, 0, 0},
new float[] {0, 0, brightness, 0, 0},
new float[] {0, 0, 0, 1, 0},
new float[] {0.1f, 0.1f, 0.1f, 0, 1}
};
ColorMatrix clrMatrix = new ColorMatrix(ptsArray);
ImageAttributes imgAttributes = new ImageAttributes();
imgAttributes.SetColorMatrix(clrMatrix, ColorMatrixFlag.Default, ColorAdjustType.Bitmap);
g.DrawImage(original, new Rectangle(0, 0, clickedImage.Width, clickedImage.Height), 0, 0, original.Width, original.Height, GraphicsUnit.Pixel, imgAttributes);
}
return clickedImage;
}
}
}
what i have tried so far is to Override the Text property to set the default value:
[DefaultValue("REC")]
public override string Text
{
get => base.Text;
set => base.Text = value;
}
public CustomButton()
{
this.FlatStyle = FlatStyle.Flat;
this.FlatAppearance.BorderSize = 0;
this.FlatAppearance.BorderColor = Color.FromArgb(0, 255, 255, 255);
this.FlatAppearance.MouseDownBackColor = Color.FromArgb(0, 255, 255, 255);
this.FlatAppearance.MouseOverBackColor = Color.FromArgb(0, 255, 255, 255);
this.DisplayFocusCues = false;
_originalImage = ResizeImage(Properties.Resources.pngimg_com___buttons_PNG152, 160, 160);
this.Size = new Size(160, 160);
this.Image = _originalImage;
// Set the default text here
if (string.IsNullOrEmpty(this.Text) || this.Text.StartsWith("customButton"))
{
this.Text = "REC";
}
_clickImage = CreateClickImage(_originalImage);
_lastImageSize = _originalImage.Size;
}
but it didn't change anything. the problem still exist.