Hello
I am sorry or not putting the framework correctly, but I cannot find WinUI
The problem is that in the canvas my points are displaying correctly
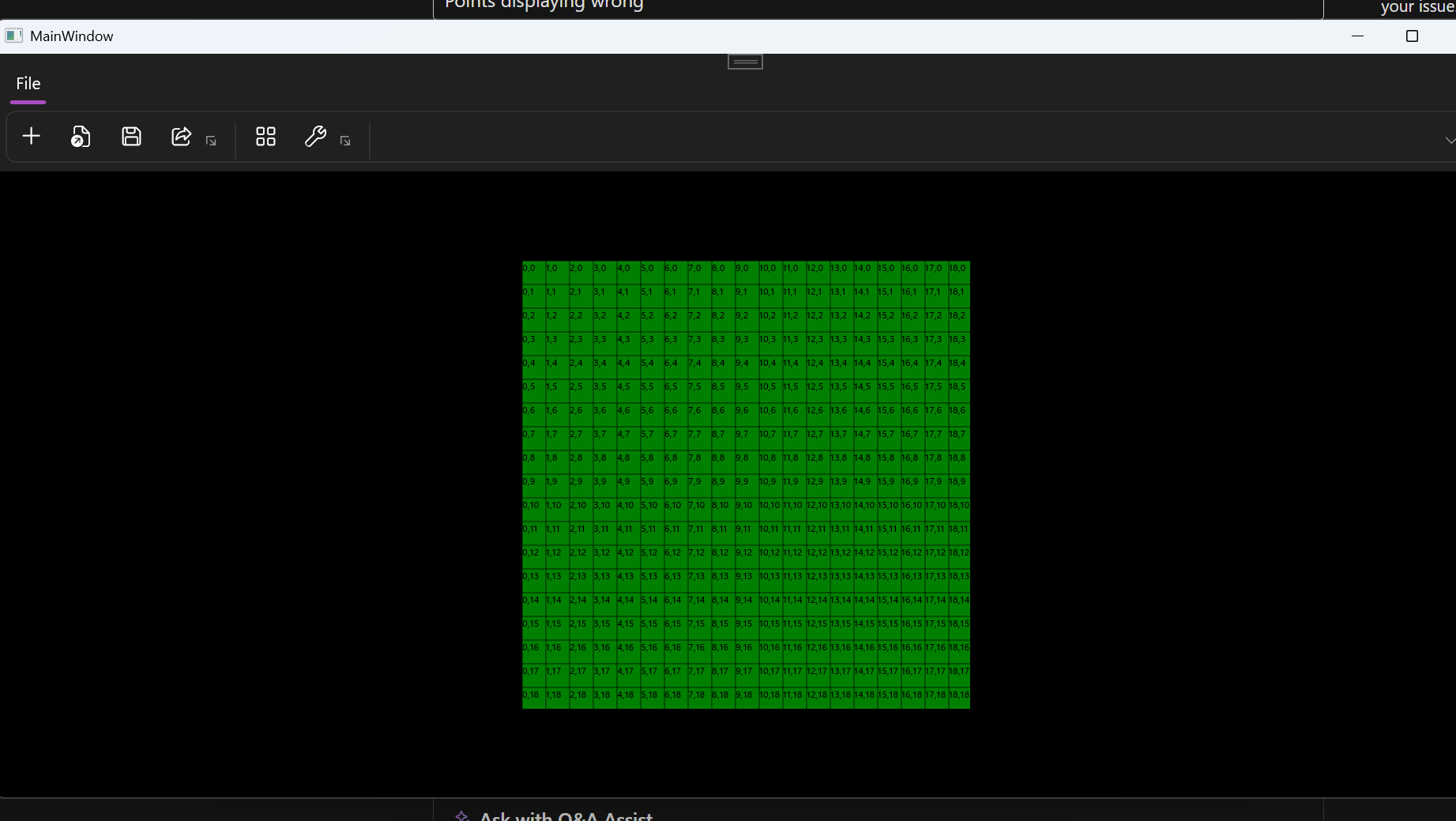
but when I go into guided mode to select my points
they dont correspond
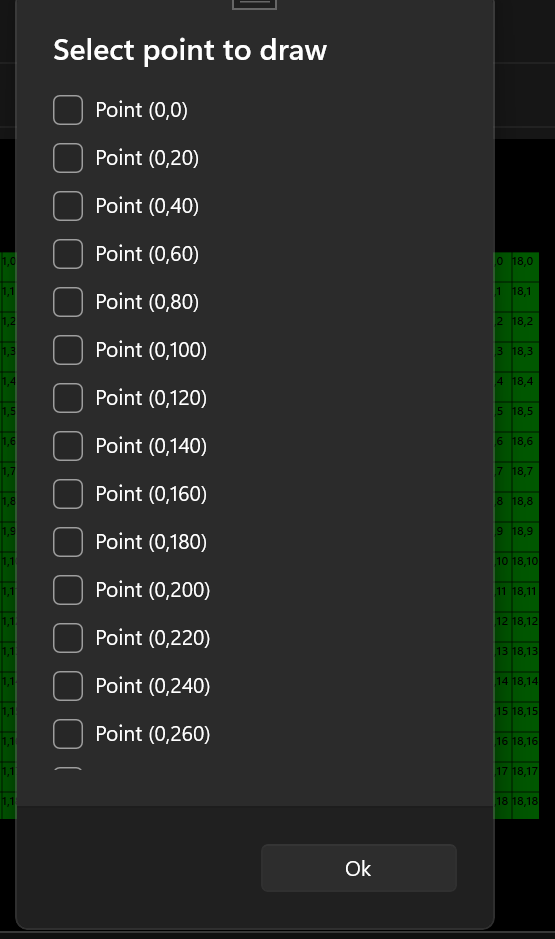
I don't have point 1, 260
my last point is two, 18,18
this is my viewModel
using System;
namespace NanoFlow.ViewModels {
public partial class MainViewModel : ObservableObject {
public ObservableCollection<PointModel> Points { get; } = [];
public ObservableCollection<LineModel> Lines { get; } = [];
private const string GRIDLINETAG = "gridLine";
private const string HELPER_TEXT = "gridText";
private Grid? _rootContainer;
private Canvas? _canvas;
private PointModel? _previousPoint;
private bool _isGridShown;
[ObservableProperty]
bool _canShowGrid;
public void SetRootContainer(object sender, RoutedEventArgs e) {
_rootContainer = sender as Grid;
}
[RelayCommand]
void CreateNewDesign() {
DrawCanvas();
CanShowGrid = true;
_canvas!.PointerPressed += Canvas_PointerPressed;
_canvas.PointerMoved += Canvas_PointerMoved;
_canvas.PointerReleased += Canvas_PointerReleased;
if(_isGridShown) {
AddGridLines(_canvas); // Pass canvas to AddGridLines
}
}
[RelayCommand]
void ToggleGridVisibility() {
_isGridShown = !_isGridShown;
if(_isGridShown) {
AddGridLines(_canvas!); // Pass canvas to AddGridLines
}
else {
RemoveGridLines();
}
}
[RelayCommand]
async Task GuidedProcessAsync() {
Points.Clear();
DrawCanvas();
AddGridLines(_canvas!); // Pass canvas to AddGridLines
ContentDialog dlg = new() {
XamlRoot = _rootContainer!.XamlRoot,
Title = "Select point to draw",
CloseButtonText = "Ok"
};
StackPanel panel = new() {
Orientation = Orientation.Vertical
};
var checkboxes = new List<CheckBox>();
foreach(var point in Points) {
var checkBox = new CheckBox {
Content = $"Point ({point.X},{point.Y})",
IsChecked = false, // Default to unchecked
Tag = point
};
panel.Children.Add(checkBox);
checkboxes.Add(checkBox);
}
dlg.Content = panel;
await dlg.ShowAsync();
}
private void AddGridLines(Canvas canvas) {
if(canvas != null) {
int gridSpacing = 20; // Spacing between grid lines in pixels
// Add vertical lines
for(int i = 0; i < canvas.Width; i += gridSpacing) {
var verticalLine = new Line {
X1 = i,
Y1 = 0,
X2 = i,
Y2 = canvas.Height,
Stroke = new SolidColorBrush(Colors.Black),
StrokeThickness = 0.5,
Tag = GRIDLINETAG
};
canvas.Children.Add(verticalLine);
}
// Add horizontal lines
for(int j = 0; j < canvas.Height; j += gridSpacing) {
var horizontalLine = new Line {
X1 = 0,
Y1 = j,
X2 = canvas.Width,
Y2 = j,
Stroke = new SolidColorBrush(Colors.Black),
StrokeThickness = 0.5,
Tag = GRIDLINETAG
};
canvas.Children.Add(horizontalLine);
}
// Add labels at the intersection points
for(int i = 0; i < canvas.Width; i += gridSpacing) {
for(int j = 0; j < canvas.Height; j += gridSpacing) {
AddGridLabel(canvas, i, j, gridSpacing); // Pass canvas to AddGridLabel
Points.Add(new PointModel(i, j));
}
}
}
}
private void DrawCanvas() {
_canvas = new Canvas {
Background = new SolidColorBrush(Color.FromArgb(255, 0, 128, 0)),
Width = 378,
Height = 378,
};
_rootContainer!.Children.Add(_canvas);
Grid.SetRow(_canvas, 1);
}
private void AddGridLabel(Canvas canvas, int x, int y, int gridSpacing) {
var textBlock = new TextBlock {
Text = $"{x / gridSpacing},{y / gridSpacing}",
FontSize = 8,
HorizontalAlignment = HorizontalAlignment.Center,
Foreground = new SolidColorBrush(Colors.Black),
Tag = HELPER_TEXT
};
// Add the tooltip to the TextBlock
AddTooltip(textBlock, x, y, gridSpacing);
Canvas.SetLeft(textBlock, x);
Canvas.SetTop(textBlock, y);
canvas.Children.Add(textBlock);
}
private void AddTooltip(TextBlock textBlock, int x, int y, int gridSpacing) {
ToolTip toolTip = new() {
Content = $"{x / gridSpacing},{y / gridSpacing}"
};
ToolTipService.SetToolTip(textBlock, toolTip);
}
private void RemoveGridLines() {
if(_canvas != null) {
// Remove grid lines
var gridLines = _canvas.Children.OfType<Line>()
.Where(l => l.Tag as string == GRIDLINETAG).ToList();
foreach(var line in gridLines) {
_canvas.Children.Remove(line);
}
// Remove grid text
var gridTexts = _canvas.Children.OfType<TextBlock>()
.Where(t => t.Tag as string == HELPER_TEXT).ToList();
foreach(var text in gridTexts) {
_canvas.Children.Remove(text);
}
}
}
private void DrawLine(PointModel startPoint, PointModel endPoint) {
var line = new Line {
X1 = startPoint.X,
Y1 = startPoint.Y,
X2 = endPoint.X,
Y2 = endPoint.Y,
Stroke = new SolidColorBrush(Colors.White),
StrokeThickness = 2,
};
_canvas!.Children.Add(line);
Lines.Add(new LineModel(startPoint, endPoint));
}
private void Canvas_PointerReleased(object sender, PointerRoutedEventArgs e) {
_previousPoint = null;
}
private void Canvas_PointerMoved(object sender, PointerRoutedEventArgs e) {
if(_previousPoint != null && e.Pointer.IsInContact) {
var position = e.GetCurrentPoint(_canvas).Position;
var currentPoint = new PointModel(position.X, position.Y);
DrawLine(_previousPoint, currentPoint);
_previousPoint = currentPoint;
}
}
private void Canvas_PointerPressed(object sender, PointerRoutedEventArgs e) {
var position = e.GetCurrentPoint(_canvas).Position;
var currentPoint = new PointModel(position.X, position.Y);
_previousPoint = currentPoint;
Points.Add(currentPoint);
var ellipse = new Ellipse {
Width = 4,
Height = 4,
Fill = new SolidColorBrush(Colors.Red)
};
Canvas.SetLeft(ellipse, currentPoint.X - 2);
Canvas.SetTop(ellipse, currentPoint.Y - 2);
_canvas!.Children.Add(ellipse);
// Connect to the previous point with a line if there is one
if(Points.Count > 1) {
var previousPoint = Points[^2];
DrawLine(previousPoint, currentPoint);
}
}
}
}
and I don't know how to display the points without the grid helping me
the grid or lines is only supposed to show when I press the
ToggleGridVisibility