Hi @Kuler Master
can I have multiple EditForms in a single razor compenent/page?
Yes, you can use multiple edit forms in a single Razor component/page. It will validate each form separately and you can access the data of different forms in the OnValidSubmit
method.
Refer to the following sample code:
@page "/multiple-forms"
@using System.ComponentModel.DataAnnotations
<h3>Form 1</h3>
<EditForm Model="@form1Model" OnValidSubmit="HandleSubmitForm1">
<DataAnnotationsValidator />
<ValidationSummary />
<div>
<label>Field 1:</label>
<InputText id="field1" @bind-Value="form1Model.Field1" />
<ValidationMessage For="@(() => form1Model.Field1)" />
</div>
<button type="submit">Submit Form 1</button>
</EditForm>
<h3>Form 2</h3>
<EditForm Model="@form2Model" OnValidSubmit="HandleSubmitForm2">
<DataAnnotationsValidator />
<ValidationSummary />
<div>
<label>Field 2:</label>
<InputText id="field2" @bind-Value="form2Model.Field2" />
<ValidationMessage For="@(() => form2Model.Field2)" />
</div>
<button type="submit">Submit Form 2</button>
</EditForm>
@code {
private Form1Model form1Model = new();
private Form2Model form2Model = new();
private void HandleSubmitForm1()
{
// Process form 1 data
}
private void HandleSubmitForm2()
{ // Access form1Model data here
var field1Data = form1Model.Field1;
// Process form 2 data along with form 1 data if needed
}
public class Form1Model
{
[Required]
public string Field1 { get; set; }
}
public class Form2Model
{
[Required]
public string Field2 { get; set; }
}
}
The result as below:
After submitting form 1:
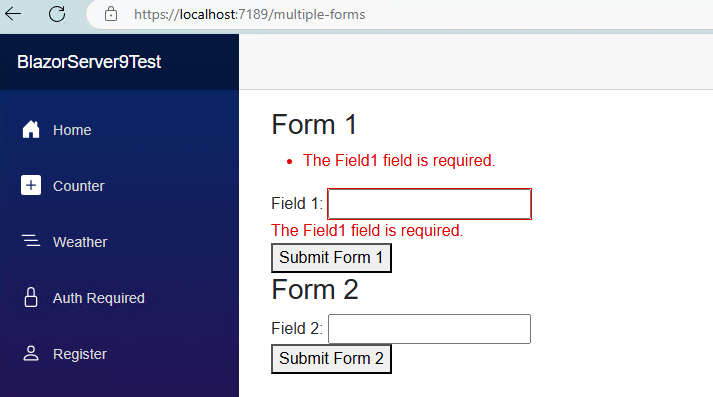
After submitting form 2:
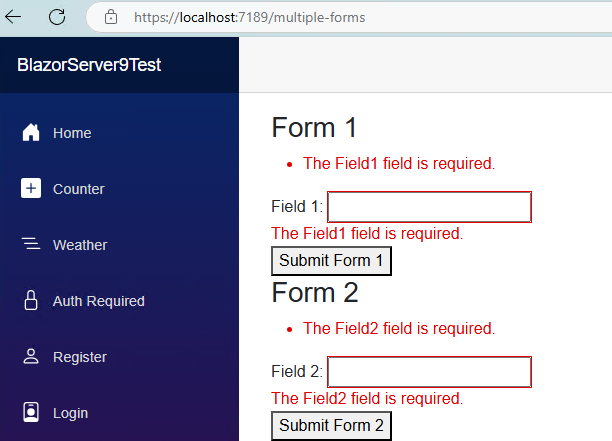
And we can get the form1 data in the Form2's OnValidSubmit
method:
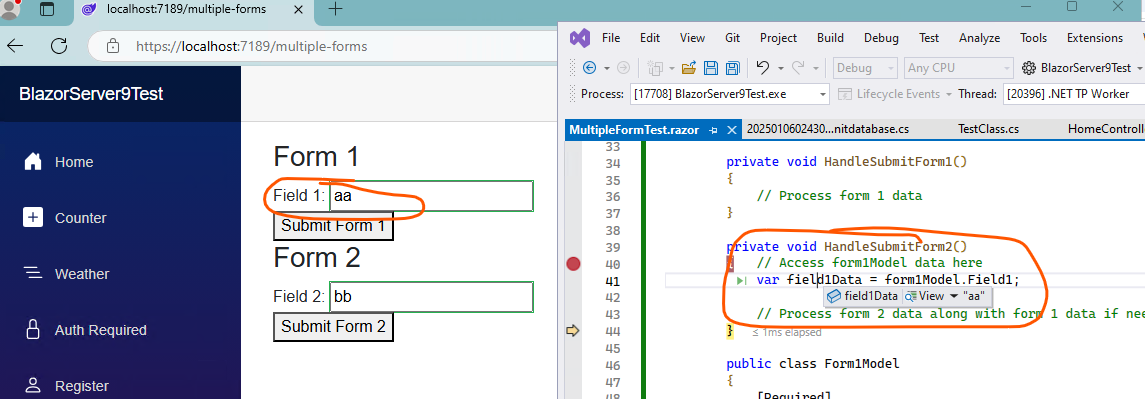
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.
Best regards,
Dillion