Hi,
I am having the below graph apis access for one note.
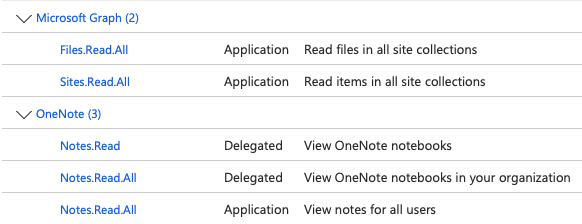
And Now I am trying to get the one note content using below code
import requests
from env import *
class SharePointNotebookLister:
def __init__(self, tenant_id, client_id, client_secret):
self.tenant_id = tenant_id
self.client_id = client_id
self.client_secret = client_secret
self.access_token = None
def get_access_token(self):
"""Get access token using MSAL"""
base_url = f"https://login.microsoftonline.com/{self.tenant_id}/oauth2/v2.0/token"
headers = {'Content-Type': 'application/x-www-form-urlencoded'}
body = {
'grant_type': 'client_credentials',
'client_id': self.client_id,
'client_secret': self.client_secret,
'scope': 'https://graph.microsoft.com/.default'
}
response = requests.post(base_url, headers=headers, data=body)
self.access_token = response.json().get('access_token')
return self.access_token
def list_site_notebooks(self, site_id=None):
"""List all notebooks in a SharePoint site"""
if not self.access_token:
self.get_access_token()
headers = {
'Authorization': f'Bearer {self.access_token}',
'Content-Type': 'application/json'
}
# If site_id is not provided, get the root site
site_relative_url = "{domain}:/sites/{site name}/" ## using right domain name and site_name
if not site_id:
site_response = requests.get(
f'https://graph.microsoft.com/v1.0/sites/{site_relative_url}',
headers=headers
)
site_response.raise_for_status()
site_id = site_response.json()['id']
# Get all notebooks in the site
notebooks_url = f'https://graph.microsoft.com/v1.0/sites/{site_id}/onenote/notebooks'
response = requests.get(notebooks_url, headers=headers)
response.raise_for_status()
notebooks = response.json().get('value', [])
return notebooks
def get_notebook_sections(self, notebook_id):
"""Get all sections in a specific notebook"""
if not self.access_token:
self.get_access_token()
headers = {
'Authorization': f'Bearer {self.access_token}',
'Content-Type': 'application/json'
}
sections_url = f'https://graph.microsoft.com/v1.0/sites/{notebook_id}/onenote/notebooks/sections'
response = requests.get(sections_url, headers=headers)
response.raise_for_status()
sections = response.json().get('value', [])
return sections
# Usage example
if __name__ == "__main__":
try:
# Initialize the notebook lister
lister = SharePointNotebookLister(tenant_id, client_id, client_secret)
access_token = lister.get_access_token()
# List all notebooks
notebooks = lister.list_site_notebooks()
print("\nFound notebooks:")
for notebook in notebooks:
print(f"\nNotebook: {notebook['displayName']}")
print(f"ID: {notebook['id']}")
print(f"Created: {notebook['createdDateTime']}")
print(f"Last Modified: {notebook['lastModifiedDateTime']}")
# Get sections for each notebook
sections = lister.get_notebook_sections(notebook['id'])
if sections:
print("\nSections:")
for section in sections:
print(f"- {section['displayName']}")
except Exception as e:
print(f"Error: {str(e)}")
I am getting this error
Error: 401 Client Error: Unauthorized for url: https://graph.microsoft.com/v1.0/sites/sparkflowsai.sharepoint.com,a783db0b-XXX-XXXX-XXXX-XXXXXXX,d8c375f6-XXXX-XXXXX-XXXX-XXXXXXX/onenote/notebooks
I am having all my notebooks inside that site and I am trying to access those note books inside that group using site_id.
Can anyone please help me to rectify where I am doing the wrong thing?