Hello Varma,
If your requirement is to generate access token and use it in PowerShell script for checking user's group membership, you can follow the steps below:
Initially, register one Microsoft Entra ID application and add below API permissions of Application type with admin consent:

In my case, I have one user named Sri as a member of Global-Admins
group:

You can make use of below PowerShell script to generate access token and check user's group membership:
function Get-Token {
$TenantID = "tenantId"
$ClientID = "appId"
$ClientSecret = "secretValue"
$TokenUri = "https://login.microsoftonline.com/$TenantID/oauth2/v2.0/token"
$Body = @{
client_id = $ClientID
client_secret = $ClientSecret
scope = "https://graph.microsoft.com/.default"
grant_type = "client_credentials"
}
try {
$Response = Invoke-RestMethod -Method Post -Uri $TokenUri -Body $Body
return $Response.access_token
}
catch {
return $null
}
}
function Is-UserInAADGroup {
param ([string]$UserEmail)
$aadtoken = Get-Token
if (-not $aadtoken) {
return $false
}
$GraphUri = "https://graph.microsoft.com/v1.0/users/$UserEmail/transitiveMemberOf"
$Headers = @{ Authorization = "Bearer $aadtoken"; "Content-Type" = "application/json" }
$AdminGroups = @("Global-Admins", "Security-Admins") | ForEach-Object { $_.ToLower().Trim() }
$AllGroups = @()
try {
do {
$Response = Invoke-RestMethod -Uri $GraphUri -Headers $Headers -Method Get
$AllGroups += $Response.value
$GraphUri = $Response.'@odata.nextLink'
} while ($GraphUri)
foreach ($Group in $AllGroups) {
if ($Group.PSObject.Properties["displayName"] -and $Group.displayName) {
$GroupName = $Group.displayName.Trim().ToLower()
if ($Group."@odata.type" -eq "#microsoft.graph.group" -and ($AdminGroups -contains $GroupName)) {
Write-Host "User: $UserEmail is a member of group $($Group.displayName), skipping deletion."
return $true
}
}
}
return $false
}
catch {
return $false
}
}
$UserEmail = "******@xxxxxxxxxxx.onmicrosoft.com"
$IsAdmin = Is-UserInAADGroup -UserEmail $UserEmail
if ($IsAdmin) {
Write-Host "$UserEmail is an admin."
} else {
Write-Host "$UserEmail is not an admin."
}
Response:

Please do not forget to "Accept the answer” and “up-vote” wherever the information provided helps you, this can be beneficial to other community members.
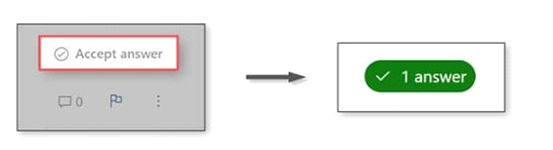
If you have any other questions or are still running into more issues, let me know in the "comments" and I would be happy to help you.